We will learn about
- Interaction with user (alert, prompt)
- Conditional Statements
- Ternary Operator (short hand if-else)
- Switch Case
Interaction with user
Alert
It shows a pop up message every time a page reloads and waits for the user to press “OK”.
Code:
alert("Welcome to my blog");
// displays a message on screen
Output:
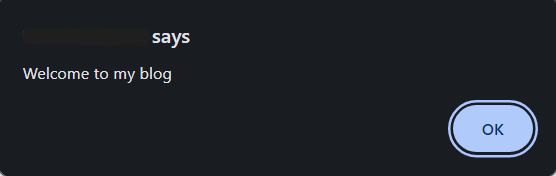
Prompt
It shows a pop up message, an input field for the visitor, and the buttons OK/Cancel.
Code:
let result = prompt("What is your age?");
console.log(result)
The visitor can type something in the prompt input field and press OK. Then we get that text in the result
. Or they can cancel the input by pressing Cancel or hitting the Esc key, then we get null
as the result
.
Output:
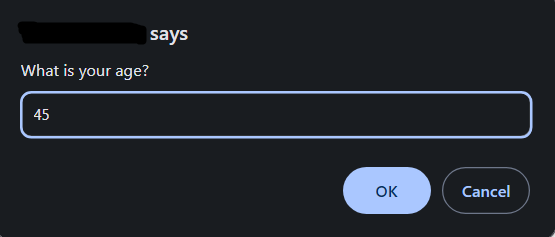
Your age is 45
Conditional Statements
While writing a program, there may be a situation when you need to adopt one out of a given set of paths. In such cases, you need to use conditional statements that allow your program to make correct decisions and perform right actions.
JavaScript supports the following forms of if..else statement −
- if statement
- if…else statement
- if…else if… statement.
if statement
The if statement is the fundamental control statement that allows JavaScript to make decisions and execute statements conditionally.
Syntax:
The syntax for a basic if statement is as follows −
if (expression) {
Statement(s) to be executed if expression is true
}
Here a JavaScript expression is evaluated. If the resulting value is true, the given statement(s) are executed. If the expression is false, then no statement would be executed. Most of the times, you will use comparison operators while making decisions.
Let’s take an example
Code:
let age = 25;
if (age > 18) {
console.log("Eligible to vote.")
}
If the condition (age > 18) is true. It will display that you are eligible to vote. Otherwise it will not show anything.
Output:
Eligible to vote.
Let’s take another example of browser.
Code:
let mode = "light";
let color;
if (mode === "dark") {
color = "black";
}
if (mode === "light") {
color = "white";
}
console.log("Color of browser is ", color);
This code will show the color of browser based on the mode. Initially the browser is in light mode (let mode = "light";)
. It will make decision whether the mode is light or dark and show us the color.
Since the mode of browser is set to light. The condition (mode === "dark")
of first if statement will become false. The program will move to next if statement.
The condition (mode === "light")
of second if statement will be checked and it is true. Code block of second if statement will be executed and color = "white"
will set the value of color to white. This line console.log("Color of browser is ", color);
prints the message.
Output:
Color of browser is white
if…else statement
The ‘if…else’ statement is the next form of control statement that allows JavaScript to execute statements in a more controlled way.
Syntax
if (expression) {
Statement(s) to be executed if expression is true
} else {
Statement(s) to be executed if expression is false
}
Here JavaScript expression is evaluated. If the resulting value is true, the given statement(s) in the ‘if’ block, are executed. If the expression is false, then the given statement(s) in the “else” block are executed. “else” does not need condition to evaluate.
Let’s take an example
Code:
let age = 15;
if (age > 18) {
console.log("Eligible to vote.")
} else {
console.log("Not eligible to vote.")
}
If the condition (age > 18) is true. It will display that you are eligible to vote. Here, age is 15 so the condition (age > 18) becomes false. else statement will be executed.
Output:
Not eligible to vote.
if…else if… statement
The if…else if… statement is an advanced form of if…else that allows JavaScript to make a correct decision out of several conditions.
Syntax
if (expression 1) {
Statement(s) to be executed if expression 1 is true
} else if (expression 2) {
Statement(s) to be executed if expression 2 is true
} else if (expression 3) {
Statement(s) to be executed if expression 3 is true
} else {
Statement(s) to be executed if no expression is true
}
It is just a series of if statements, where each if is a part of the else clause of the previous statement. Statement(s) are executed based on the true condition, if none of the conditions is true, then the else block is executed.
Code:
let age = 45;
if (age < 18) {
console.log("Junior");
} else if (age < 60) {
console.log("Middle");
} else {
console.log("Senior");
}
Age is 45. First if condition (age < 18) becomes false. It will check the next else if condition (age < 60). This condition becomes true so it will display “Middle”. Once a condition becomes true, the relevant statement is executed. The program will not check further if a condition becomes true and the program ends.
Output:
Middle
Ternary Operator (Short Hand If…Else)
There is also a short-hand if else, which is known as the ternary operator because it consists of three operands. It can be used to replace multiple lines of code with a single line. It is often used to replace simple if else statements:
Syntax
(condition) ? expression1 : expression2;
If condition is true, expression 1 will be executed. If condition is false, expression 2 will be executed.
Instead of writing
Code:
let age = 15;
if (age > 18) {
console.log("Eligible to vote.")
} else {
console.log("Not eligible to vote.")
}
We can write
Code:
let age = 15;
let result = age > 18 ? "adult" : "not adult";
console.log(result);
The expression (age > 18 ? "adult" : "not adult")
will return some value. We will store the value in a variable named result. Since age is not greater than 18, the condition (age > 18)
becomes false. So the second expression not adult
will be returned and stored in result
. The value of result will then be printed.
Output:
not adult
“switch” statement
A switch
statement can replace multiple if-else-if
checks.
It gives a more descriptive way to compare a value with multiple variants.
Syntax
switch (expression) {
case condition 1:
statement(s) to be executed
break;
case condition 2:
statement(s) to be executed
break;
...
case condition n:
statement(s) to be executed
break;
default:
statement(s)
}
Lets take an example of grades.
Code:
let grade = "C";
switch (grade) {
case "A":
console.log("Good job");
break;
case "B":
console.log("Pretty good");
break;
case "C":
console.log("Passed");
break;
case "D":
console.log("Not so good");
break;
case "F":
console.log("Failed");
break;
default:
console.log("Unknown grade");
}
- Since
grade
has the value “C”, theswitch
statement starts by evaluating the firstcase
block, which checks ifgrade
is equal to “A”. - As “C” is not equal to “A”, the code in this
case
block is skipped. - The evaluation continues down the
case
blocks until it reaches the block that checks ifgrade
is equal to “C”. - Because “C” matches the value of
grade
, the code in thiscase
block is executed:console.log("Passed");
prints the message “Passed” to the console.
- The
break
statement after this line ensures that the code doesn’t proceed to any furthercase
blocks, even ifgrade
might match them as well.
Default Case:
- If the value of
grade
doesn’t match any of the specifiedcase
values, the code falls through to thedefault
block. - In this case, the code
console.log("Unknown grade");
would be executed, printing the message “Unknown grade” to the console.
Output:
Passed
Leave a Reply